http://www.systemverilog.in/forum/showthread.php?tid=143
Friday, November 23, 2012
Post 14 : Introduction to OOPs
OOP Can be described with following concepts :
- Follows bottom up approach.
- Emphasis is on data. //need to search explanation
- Programs are divided into objects.
- Functions and data are bound together.
- Communication is done through objects.
- Data is hidden.
Classes, Objects, Data abstraction and encapsulation, Polymorphism, Inheritance, Message Passing, and Dynamic Binding.
1. What is a class?
Class is an entity which consists of member data and member functions which operate on the member data bound together.
2. What is an object?
i)Objects are instances of classes.
ii) Class is a collection of similar kind of objects.
iii) When a class is created it doesn’t occupy any memory
iv) but when instances of class is created i.e., when objects are created they occupy memory space.
3. What is encapsulation?
Encapsulation is welding of code and data together into objects.
4. What is inheritance?
Inheritance is a mechanism through which a subclass inherits the properties and behavior of its superclass.
The derived class inherits the properties and method implementations of the base class and extends it by overriding methods and adding additional properties and methods.
5. What is polymorphism?
i) In Greek this means "many shapes."
ii) As a consequence of inheritance and virtual functions, a single task (for example, drawing a geometrical shape) can be implemented using the same name (like draw()) and implemented differently (via virtual functions) as each type in object hierarchy requires(circle.draw() or rectangle.draw()).
iii) Later, when a polymorphic object (whose type is not known at compile time) executes the draw() virtual function, the correct implementation is chosen and executed at run time.
6. What is the difference between function overloading and function overriding?
i) Overloading is a method that allows defining multiple member functions with the same name but different signatures.
The compiler will pick the correct function based on the signature.
ii) Overriding is a method that allows the derived class to redefine the behavior of member functions which the derived class inherits from a base class.
The signatures of both base class member function and derived class member function are the same; however, the implementation and, therefore, the behavior will differ.
7. What are the advantages of OOP?
- Data hiding helps create secure programs.
- Redundant code can be avoided by using inheritance.
- Multiple instances of objects can be created.
- Work can be divided easily based on objects.
- Inheritance helps to save time and cost.
- Easy upgrading of systems is possible using object oriented systems.
8. Explain about the virtual task and methods .
#important : Virtual tasks and functions are the ways to achieve
the polymorphism in system verilog.Example :
01.
class
base ;
03.
virtual
function
int
print;
04.
$display(
"INSIDE BASE \n"
);
05.
endfunction : print
06.
07.
endclass
: base
08.
09.
class
derived
extends
base;
10.
11.
function
int
print;
12.
$display(
"INSIDE DERIVED \n"
);
13.
endfunction : print
14.
15.
endclass
: derived
16.
17.
18.
19.
program test ;
20.
21.
derived d1;
22.
initial
23.
begin
24.
d1 =
new
();
25.
d1.print();
26.
callPrint (d1);
27.
end
28.
29.
task
callPrint (base b1);
30.
$display(
"Inside callPrint \n"
);
31.
b1.print;
32.
endtask
: callPrint
33.
34.
endprogram
35.
36.
Output :
37.
========
38.
39.
VSIM 1> run
40.
# INSIDE DERIVED
41.
#
42.
# Inside callPrint
43.
#
44.
# INSIDE BASE
45.
#
Subscribe to:
Posts (Atom)
Ethernet and more
Ethernet is a protocol under IEEE 802.33 standard User Datagram Protocol (UDP) UDP is a connectionless transport protocol. I...
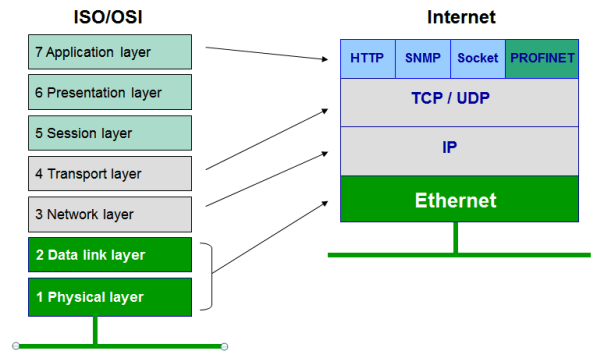
-
Amba AXI is targeted at high performance, suitable for high-speed submicron connect. Features: 1.separate address/control and dat...
-
From the starting of simulation till the end of the simulation: Why Phases Concept : 1. In order to have a consistent Testbench flow, ...
-
The reason there are two macros is because the factory design pattern fixes the number of arguments that a constructor can have. Classes de...